Some old database still store data in ascii characters. However, today's website is all unicode or utf enabled. That means it's able to support more characters (such as Japanese, French, Chinese and etc).
using System.Text;
private string ConvertUnicodeToAnsi(string input)
{
if (input.Length == 0) return string.Empty;
var ascii = new ASCIIEncoding();
return ascii.GetString(ascii.GetBytes(input));
}
Monday, November 23, 2009
SQL2008 Intellisense Quick Fix
I opened a query window under SQL2008 Management studio. Typing "Select * from " , presses ctrl+space bar to bring up the table intellisense. It's not displaying the table in the list (although I have ran the use command on a database).
What have I done?
What are the work-arounds?
1. Use full schema in select definition:
Type select * from AdventureWorks.dbo.tblApplication
2.Work-around I found by accident.
Leave the use command on the first line. Type select * from" and ctrl+space. It shows tblApplication in the intellisense result.
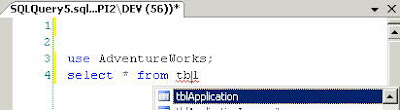
What have I done?
- use AdventureWorks; // this connect my query window the database I wish to use.
- Type select * from + [Ctrl+ space bar]. My table that resides in the AdventureWorks db doesn't exist in the drop-down list (as shown below, the list doesn't show tblApplication).
What are the work-arounds?
1. Use full schema in select definition:
Type select * from AdventureWorks.dbo.tblApplication
2.Work-around I found by accident.
Leave the use
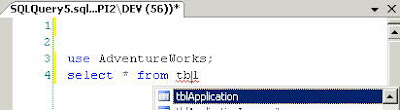
Saturday, October 31, 2009
Comodo Firewall - Remote desktop rule
Scenario
I have upgraded Comodo Firewall to a new version recently. Unfortunately, I haven't been able to remote desktop to my work pc since.
Ruled out vpn settings and no http tunnelling on remote desktop. The remote desktop works if the firewall is turned OFF. This sounds like firewall has the remote desktop port blocked.
Bingo. The problem lies in the remote desktop port 3389 is blocked by Comodo Firewall.
What to do in Comodo firewall?
I have upgraded Comodo Firewall to a new version recently. Unfortunately, I haven't been able to remote desktop to my work pc since.
Ruled out vpn settings and no http tunnelling on remote desktop. The remote desktop works if the firewall is turned OFF. This sounds like firewall has the remote desktop port blocked.
Bingo. The problem lies in the remote desktop port 3389 is blocked by Comodo Firewall.
Resolution
- Add a firewall rule to allow TCP stream coming through port 3389. Voila, everything is back to normal.What to do in Comodo firewall?
- Open the firewall application. Click on the Firewall tab (next to Summary tab).
- On the Firewall tasks, select Advance.
- Click on Network Security Policy. You will see a window with 2 tabs appears. Application Rules and Global Rules.
- Click on Global rules, click Add to bring up Network Control Rule dialog.
- Action: Allow
- Protocol: TCP
- Direction: In
- Description: type the label for this rule. i.e. Remote desktop 3389
- Source Address/Destination Address/Source Port: Any
- Destination port: a single port. Port: 3389.
- Click Apply to add the rule.
- Select the newly added rule from the Global Rules listing and move it to the top. This is to make sure our new rule is given the highest priority.
- Done. Why not try it now.
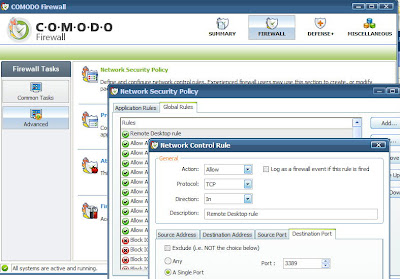
Tuesday, October 27, 2009
British date time regular expression dd/mm/yyyy
I came up with the following before which is not entirely correct.
^\d{2}/(\d{2})/(\d{4})+
Why? You can enter the date as 45\23\0088. This is just digit matching.
thus, i refactor to the following:
((0{1}[1-9])|([1-2][0-9])|(3{1}[0-1]))/((0{1}[1-9])|(1{1}[0-2]))/((19|20)\d\d)
This regex only allow you to enter
dd from 01-31.
mm from 01-09 and 11-12
yyyy range restricted to 19xx-20xx.
How to get it tested? Try the following flash regex engine:
http://gskinner.com/RegExr/

^\d{2}/(\d{2})/(\d{4})+
Why? You can enter the date as 45\23\0088. This is just digit matching.
thus, i refactor to the following:
((0{1}[1-9])|([1-2][0-9])|(3{1}[0-1]))/((0{1}[1-9])|(1{1}[0-2]))/((19|20)\d\d)
This regex only allow you to enter
dd from 01-31.
mm from 01-09 and 11-12
yyyy range restricted to 19xx-20xx.
How to get it tested? Try the following flash regex engine:
http://gskinner.com/RegExr/

Friday, October 09, 2009
How to terminate remote desktop session
List the session id on the host server
qwinsta /server:[host]
Terminate the specific rdp session on the host
rwinsta [sessionID] /server:[host]
qwinsta /server:[host]
Terminate the specific rdp session on the host
rwinsta [sessionID] /server:[host]
Saturday, September 05, 2009
LlblGen - ORM tool
What is Llblgen?
It is a tool that generate data access layer from your database . Thus, saving developer time you on writing CRUD (create, retreive ,update, delete) code in the .net framework.
Feature list:
- Multiple databases support. MsSQL, Oracle, MySQL, MS Access and etc
- All .net framework version support. This include compact framework.
- Most database access operation is supported. Table record operation, retrieve views and store procedure execution.
- Linq is supported.
- DAL supports both desktop and window application development.
I am still not impressed, who is using it?
Check the company list here
Read more about Llblgen Pro
It is a tool that generate data access layer from your database . Thus, saving developer time you on writing CRUD (create, retreive ,update, delete) code in the .net framework.
Feature list:
- Multiple databases support. MsSQL, Oracle, MySQL, MS Access and etc
- All .net framework version support. This include compact framework.
- Most database access operation is supported. Table record operation, retrieve views and store procedure execution.
- Linq is supported.
- DAL supports both desktop and window application development.
I am still not impressed, who is using it?
Check the company list here
Read more about Llblgen Pro
Where to download Northwind database?
I am working with Llblgen recently. The sample code requires the SQL2000 Northwind database. It took me some time to locate it. Let share the link then:
Download Northwind database samples
The sample installs the samples on 'C:\SQL Server 2000 Sample Databases' folder.
How about just download Northwind sql script?
Here you go . Rename the file to instnwnd.sql after download.
Is the script compatible with other version of SQL server (SQL2005, SQL2008) ?
Yes. Use the instnwnd.sql. Execute the sql script in your SQL Management Studio. The script create the Northwind database schemas with sample data. At least database backup (mdf and ldf) compatibility is least of your problem.
I didn't try the mdf backup restore but did create the db in SQL2008 instance using just sql script. No problem at all.
Happy trying. :)
Download Northwind database samples
The sample installs the samples on 'C:\SQL Server 2000 Sample Databases' folder.
How about just download Northwind sql script?
Here you go . Rename the file to instnwnd.sql after download.
Is the script compatible with other version of SQL server (SQL2005, SQL2008) ?
Yes. Use the instnwnd.sql. Execute the sql script in your SQL Management Studio. The script create the Northwind database schemas with sample data. At least database backup (mdf and ldf) compatibility is least of your problem.
I didn't try the mdf backup restore but did create the db in SQL2008 instance using just sql script. No problem at all.
Happy trying. :)
Monday, August 17, 2009
How to change SQL server 2008 instance name
Find out the server instance name
- sp_helpserver
- select @@servername
Modify the instance name
- sp_dropserver ‘old_name’
- go
- sp_addserver ‘new_name’,‘local’
- go
Friday, August 14, 2009
Vs2008 snippets
Snippet Designer (MSDN blog)
http://www.codeplex.com/SnippetDesigner
C# snippet library
http://snippetlibcsharp.codeplex.com/
Visual Studio snippet directory
C:\Program Files\Microsoft Visual Studio 9.0\VC#\Snippets\1033\Visual C#\
http://www.codeplex.com/SnippetDesigner
C# snippet library
http://snippetlibcsharp.codeplex.com/
Visual Studio snippet directory
C:\Program Files\Microsoft Visual Studio 9.0\VC#\Snippets\1033\Visual C#\
Friday, July 03, 2009
LINQ Example
LINQ (Language Integrated Query) is Microsoft query language that enable you to not just query your objects collection directly (List), but also XML documents, DataRow from relational database system.
Characteristics:
- Only works with supportable data source (backed by LINQ provider). LINQ to Objects|SQL|XML|Entities|DataSets.
- Deferred execution.
Imagine the following scenarios:
1. How do you select a customers with country='gb'?
var customers = new List{
new Customer(){ID=1, Name="Abba", Country="gb", Yob="1950"},
new Customer(){ID=2, Name="Bubble", Country="gb", Yob="1970"},
new Customer(){ID=3, Name="Chaplin", Country="us", Yob="1940"},
new Customer(){ID=4, Name="Gum", Country="gb", Yob="2009"},
};
Normal way:
foreach(Customer c in customers)
{
if(c.country.ToLover().Equals("gb") {...}
}
LINQ way:
var query = from c in customers
where c.country.ToLower().Equals("gb")
select c;
Verdict:
Nothing special. right?
2. What if you want to sort the results of scenario 1 by name (ASC) followed by year of birth (DESC)?
LINQ:
var query = from c in customers
where c.country.Equals("gb")
orderby c.name ascending, c.yob descending,
select c;
3. Any shortcut to scenario 2. Yes.
The LINQ query could further translate to
var query =
customers.OrderBy(s=> s.name).OrderByDescending(s=>s.yob);
4. How to find distinct word from senteces?
string[] chickenQuotes = {
" The chicken did not cross the road. This is a complete fabrication. We do not even have a chicken",
" We just want to know if the chicken is on our side of the road or not. The chicken is either with us or against us."
}
var query =
(from chickenQuotes.SelectMany(s=>s.Split(' '))
where !word.Equals('.')
select word).Distinct();
Home time.. continue later
Characteristics:
- Only works with supportable data source (backed by LINQ provider). LINQ to Objects|SQL|XML|Entities|DataSets.
- Deferred execution.
Imagine the following scenarios:
1. How do you select a customers with country='gb'?
var customers = new List
new Customer(){ID=1, Name="Abba", Country="gb", Yob="1950"},
new Customer(){ID=2, Name="Bubble", Country="gb", Yob="1970"},
new Customer(){ID=3, Name="Chaplin", Country="us", Yob="1940"},
new Customer(){ID=4, Name="Gum", Country="gb", Yob="2009"},
};
Normal way:
foreach(Customer c in customers)
{
if(c.country.ToLover().Equals("gb") {...}
}
LINQ way:
var query = from c in customers
where c.country.ToLower().Equals("gb")
select c;
Verdict:
Nothing special. right?
2. What if you want to sort the results of scenario 1 by name (ASC) followed by year of birth (DESC)?
LINQ:
var query = from c in customers
where c.country.Equals("gb")
orderby c.name ascending, c.yob descending,
select c;
3. Any shortcut to scenario 2. Yes.
The LINQ query could further translate to
var query =
customers.OrderBy(s=> s.name).OrderByDescending(s=>s.yob);
4. How to find distinct word from senteces?
string[] chickenQuotes = {
"
"
}
var query =
(from
where !word.Equals('.')
select word).Distinct();
Home time.. continue later
Thursday, June 25, 2009
Regular Expression to search for time duration
How to find the following time string in a text file?
(09:00 -12:00)
Try regular expression:
\((\d{1,2}:\d{1,2}.*\d{1,2}:\w*)\)
Imagine you are trying to remove the time from this sting "meet up with friends (09:00 - 12:00)"
Code:
string result = System.Text.RegularExpressions.Regex.Replace("meet up with friends (09:00 - 12:00)", @"\((\d{1,2}:\d{1,2}.*\d{1,2}:\w*)\)",string.Empty)
Result:
result = "meet up with friends" is produced.
(09:00 -12:00)
Try regular expression:
\((\d{1,2}:\d{1,2}.*\d{1,2}:\w*)\)
Imagine you are trying to remove the time from this sting "meet up with friends (09:00 - 12:00)"
Code:
string result = System.Text.RegularExpressions.Regex.Replace("meet up with friends (09:00 - 12:00)", @"\((\d{1,2}:\d{1,2}.*\d{1,2}:\w*)\)",string.Empty)
Result:
result = "meet up with friends" is produced.
Friday, June 12, 2009
Google Search Tips
There is plenty of little shortcuts we could produce better search results via Google.
Dictionary search
Syntax= define:
Type define: constituency for example.

Unit Conversion
Examples:
10 EUR in GBP
25 miles in km

Weather report:
tokyo weather
london weather
Search on a particular website
Syntax= site:
transformers site: www.imdb.com
Find related site
related: msdn.microsoft.com
Find pages that has links to a specific site
link:http://www.bbcnews.co.uk
Display a cache version of your website by Google
cache:www.zippie.net
Find Info about a page
info:www.fgsman.org.uk
Google group search
author:Mark Russinovich insubject:"process debugging"
Find word widgets but has to contains red or blue keyword
widgets (red OR blue)
Return results based on specific file type
intitle:j2ee visual tutorial filetype:pdf
service oriented architecture filetype:pdf site:edu
Search all words in title
allintitle: msdn .net framework 4
Search any words contains 2009 in url
intitle:msdn .net inurl:2009
Dictionary search
Syntax= define:
Type define:

Unit Conversion
Examples:
10 EUR in GBP
25 miles in km

tokyo weather
london weather
Search on a particular website
Syntax=
transformers site: www.imdb.com
Find related site
related: msdn.microsoft.com
Find pages that has links to a specific site
link:http://www.bbcnews.co.uk
Display a cache version of your website by Google
cache:www.zippie.net
Find Info about a page
info:www.fgsman.org.uk
Google group search
author:Mark Russinovich insubject:"process debugging"
Find word widgets but has to contains red or blue keyword
widgets (red OR blue)
Return results based on specific file type
intitle:j2ee visual tutorial filetype:pdf
service oriented architecture filetype:pdf site:edu
Search all words in title
allintitle: msdn .net framework 4
Search any words contains 2009 in url
intitle:msdn .net inurl:2009
Friday, June 05, 2009
How to find referenced table in MS SQL 2005?
SELECT so.name
FROM sysobjects so INNER JOIN
sysreferences sr ON so.id = sr.rkeyid
WHERE (so.type = 'U') AND (sr.fkeyid = OBJECT_ID('Customer'))
Result:
name
CustomerGroup
CustomerCountry
Rating
CustomerType
FROM sysobjects so INNER JOIN
sysreferences sr ON so.id = sr.rkeyid
WHERE (so.type = 'U') AND (sr.fkeyid = OBJECT_ID('Customer'))
Result:
name
CustomerGroup
CustomerCountry
Rating
CustomerType
How to edit a pdf file?
What do you normally use to edit a pdf file? Adobe Acrobat? Or create/edit the document in Microsoft Word and save it as pdf document?
Why not check out Foxit PdfEdit? A light weight pdf editor.
More information from this site.
Try 6 months free license by clicking on the link below.
Why not check out Foxit PdfEdit? A light weight pdf editor.
More information from this site.
Try 6 months free license by clicking on the link below.

Wikipedia or Wiktionary
Most of us know what is Wikipedia - free online encyclopedia contributed by everybody. Is there an equivalent of online dictionary? Yes. Wiktionary.
How to use it?
How to use it?
- Navigate to the site Wiktionary.
- Type in your word and the drop down appears (depicted below). Select and click search.

Thursday, June 04, 2009
How to insert code snippet in Visual Studio
Thursday, May 28, 2009
Wednesday, May 27, 2009
How to find out window environment values another way
Normally, you would show the System Properties window.
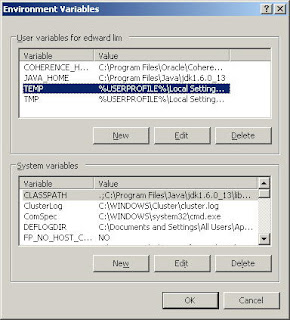
Method 1 - Navigate via MyComputer
Right click on the My computer -> Properties -> Goto Advanced tab -> Click on Environment Variables. Then see Temp = %USERPROFILE%\Local Settings\Temp
Method 2 - msinfo32
Type msinfo32 in the run command.
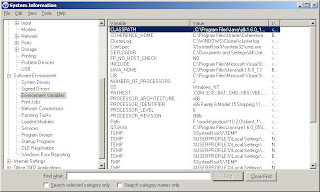
Method 3 - Dos command
Open command prompt by typing in cmd. type the following:
echo %path%
Method 4 - Powershell tool
Open powershell and type
4.1) $env:path // show envrionmen vairable ("path")
4.2) [Environment]::GetEnvironmentVariable("path")
How to show all environment vairables in powershell
Get-Item $env:* or env:*
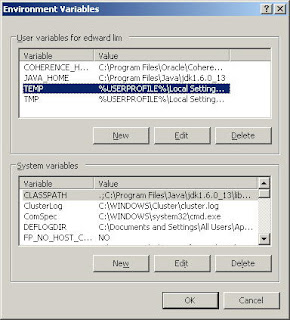
Method 1 - Navigate via MyComputer
Right click on the My computer -> Properties -> Goto Advanced tab -> Click on Environment Variables. Then see Temp = %USERPROFILE%\Local Settings\Temp
Method 2 - msinfo32
Type msinfo32 in the run command.
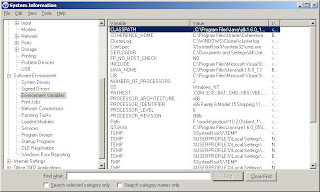
Method 3 - Dos command
Open command prompt by typing in cmd. type the following:
echo %path%
Method 4 - Powershell tool
Open powershell and type
4.1) $env:path // show envrionmen vairable ("path")
4.2) [Environment]::GetEnvironmentVariable("path")
How to show all environment vairables in powershell
Get-Item $env:* or env:*
Friday, May 22, 2009
Save and restore Powershell history session
Use this command to store powershell session history into xml file
Get-History | Export-Clixml "c:\scripts\my_history.xml"
Use this to restore Powershell history from file.
Import-Clixml "C:\PowershellSession.xml" |Add-History
How to show the list of command history?
h or Get-History
How can i find out the alias for Get-History?
Get-Alias -Definition Get-History

What to do if I have duplicate command in the history?
We wish to have a unique entry per command. Edit the window properties and check 'Discard Old Duplicates'.
Get-History | Export-Clixml "c:\scripts\my_history.xml"
Use this to restore Powershell history from file.
Import-Clixml "C:\PowershellSession.xml" |Add-History
How to show the list of command history?
h or Get-History
How can i find out the alias for Get-History?
Get-Alias -Definition Get-History

What to do if I have duplicate command in the history?
We wish to have a unique entry per command. Edit the window properties and check 'Discard Old Duplicates'.
Set command window to powershell background
Sunday, May 17, 2009
How to hide vista users at Welcome screen
- Type regedit to run Registry Editor
- Navigate to HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows NT\CurrentVersion\Winlogon\SpecialAccounts\UserList.
- Create folder for SpecialAccounts or UserList in the registry order above if it's not found. Right click on the Winlogon folder in registry editor and select new folder to create the folder.
- Create a new DWORD(32 bits for 32bits vista os) key with value 0 (as default) under UserList registry folder. Name the key as the vista login you would like to hide.
- For example, if your vista login name is Belacan. Then the new key should be belacan (case in-sensitive).
- The DWORD value 0 indicates hiding. You can set the value to 1 if you would like to show it in the future.
Disable Vista 'Need your permission to continue'
Whenever a user performs what window think it potentially risky operation, the following message is displayed to warn and asked for confirmation.
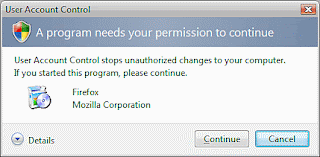
How to disable it?
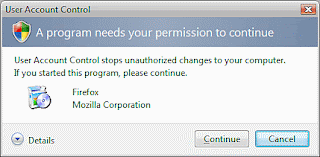
How to disable it?
- Open control panel, type user account in the search box.
- Click on 'Turn User Account Control (UAC) on or off.
- Uncheck the the tickbox and restart window.
- Voila. No more annoying pop-up.
Friday, May 15, 2009
Window Azure
Window Azure is the new buzzword recently from Microsoft. It's just a platform to host and run application at Microsoft data centres. Thus, cutting the needs to maintain and invest in your own hardware.
Load balancing, workflow, SQL data services , Live/Active Directory authentication are part of the hosting package. There is 5 services that developers can develop application on that run in the cloud - Live Services, .Net Services, SQL Services, SharePoint and Microsoft CRM.

Microsoft Definition:
The Azure™ Services Platform (Azure) is an internet-scale cloud services platform hosted in Microsoft data centers, which provides an operating system and a set of developer services that can be used individually or together.
The other benefit is scale-as-you-go. Imagine you develop an apps and released to production (deployed to Azure Services Platform). The customer response is surging and your site can't cope with the traffic.
If the apps is hosted in Window Azure platform, a flip of switch ( change of config file instance properties from 1 -> 200) will enable your app instances to increase to what is specified.

However, cloud computing is not new. Companies such as Google (Google App Engine), Amazon (Amazon S3 and Web Services) and IBM (Blue Cloud) have been offering their services for some time.
Further references:
http://www.microsoft.com/azure/windowsazurefordevelopers/Default.aspx?path=AzureWhitePapers
Azure .Net service
http://msdn.microsoft.com/en-us/azure/netservices.aspx
Load balancing, workflow, SQL data services , Live/Active Directory authentication are part of the hosting package. There is 5 services that developers can develop application on that run in the cloud - Live Services, .Net Services, SQL Services, SharePoint and Microsoft CRM.

Microsoft Definition:
The Azure™ Services Platform (Azure) is an internet-scale cloud services platform hosted in Microsoft data centers, which provides an operating system and a set of developer services that can be used individually or together.
The other benefit is scale-as-you-go. Imagine you develop an apps and released to production (deployed to Azure Services Platform). The customer response is surging and your site can't cope with the traffic.
If the apps is hosted in Window Azure platform, a flip of switch ( change of config file instance properties from 1 -> 200) will enable your app instances to increase to what is specified.

However, cloud computing is not new. Companies such as Google (Google App Engine), Amazon (Amazon S3 and Web Services) and IBM (Blue Cloud) have been offering their services for some time.
Further references:
http://www.microsoft.com/azure/windowsazurefordevelopers/Default.aspx?path=AzureWhitePapers
Azure .Net service
http://msdn.microsoft.com/en-us/azure/netservices.aspx
Monday, May 11, 2009
Crash Dump Analysis
My window crashes recently and left me a 171Mb Memory.dmp file at c:\windows. I decided to find out more about what happened. Windbg download from here.
Open WinDbg and load the symbol server from the link below: srv*c:\symbols*http://msdl.microsoft.com/download/symbols
Open Crash Dump/Ctrtl+D and open c:\windows\memory.dmp.
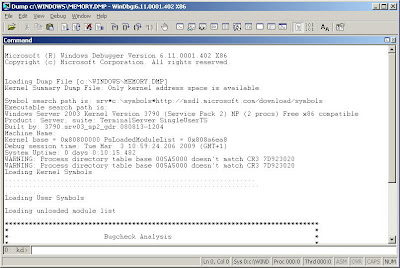
To be continued .... (quite busy recently)
Open WinDbg and load the symbol server from the link below: srv*c:\symbols*http://msdl.microsoft.com/download/symbols
Open Crash Dump/Ctrtl+D and open c:\windows\memory.dmp.
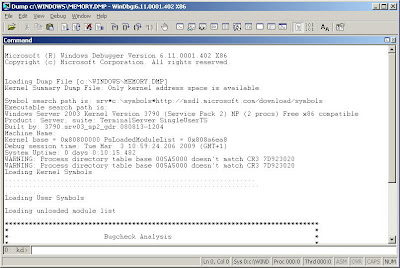
To be continued .... (quite busy recently)
Friday, May 08, 2009
C# Delegate put in use
A deeper look into delegate use just blown me away. It make the code so modular and clean that the old way of passing in object and dependencies changes issues is resolved.
What is delegate?
Delegate is a type that can associate with any method with compatible signature. It's like pointer to function.
Delegate declaration and usage
1. Declare: delegate signature(parameters)
http://msdn.microsoft.com/en-us/library/ms173176.aspx
What are the type of delegates?
1. Object type is passed in at run-time.
MSDN Delegate reference guide
http://msdn.microsoft.com/en-us/library/ms173174.aspx
Simple Direct Examples
http://www.akadia.com/services/dotnet_delegates_and_events.html
What is delegate?
Delegate is a type that can associate with any method with compatible signature. It's like pointer to function.
Delegate declaration and usage
1. Declare: delegate signature(parameters)
public delegate void ProcessBookDelegate(Book book);3. Subscriber method and Invoke: SubscriberMethod(delegate name)
2. Instantiate delegate instance and associate with handler method
bookDB.ProcessPaperbackBooks(PrintTitle);
bookDB.ProcessPaperbackBooks(totaller.AddBookToTotal);
public void ProcessPaperbackBooks(ProcessBookDelegate processBook)The above example in details. What the code achieved is call different method via a common method (depending on the which method is associated with the delegate)
{
processBook(mybook);
}
http://msdn.microsoft.com/en-us/library/ms173176.aspx
What are the type of delegates?
- Covariance - delegates can be used with methods that have return types that are derived from the return type in the delegate signature. In other words, the delegate signature can returns type of base class or inherited type.
- Contravariance - subscriber can use the same delegate to handle different method as long as the delegate signature base type is the same. A muticasting example, Base class - Vehicle has children class Truck and Car. Subscriber class -
.OnTheMove += Truck.Move + Car.Move.
1. Object type is passed in at run-time.
public delegate void DelFurther reading:(T item);
public void Notify(int i) { }
Del<int> d2 = Notify;
MSDN Delegate reference guide
http://msdn.microsoft.com/en-us/library/ms173174.aspx
Simple Direct Examples
http://www.akadia.com/services/dotnet_delegates_and_events.html
Wednesday, April 29, 2009
Window PowerShell 2 Quick Tips
Windows PowerShell
Windows PowerShell is a command-line shell and scripting environment that brings the power of the .NET Framework to command-line users and script writers. It introduces a number of powerful new concepts that enables you to extend the knowledge you have gained and the scripts you have created within the Windows Command Prompt and Windows Script Host environments.
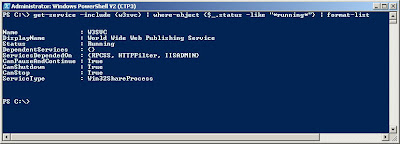
Figure 1.0 PowerShell window.
Windows PowerShell V2 Community Technology Preview 3 (CTP3)
PowerShell Benefits
I hope the following list of examples could get you playing:
1. Get help on particular command: man . E.g. man get-service
2. See a list of command available: get-command
3. Clear the screen: clear-host
4. Show info for Http Web Server service: get-service -include {w3svc} | where-object {$_.status -like "*running*"} | format-list (As in Figure 1.0 command)
5. Get the system date: get-date
6. Get the service DisplayName only: get-service w3svc | format-list -property DisplayName
7. List service : get-service | where-object {$_.name -like "[v-z]*"} | Sort -descending "Status"
8. Show .net component methods: [System.Math] | Get-Member -MemberType Methods
9. Get newest 5 system event log entry:
Get-EventLog -newest 5 -ComputerName confucius -logname System | Format-List Index, Message
10. List a service member. The following snippet filter the remote service on a machine fireserver name to httpd (Apache Web server)
"httpd"| Get-Service -computername fireserver | Get-Member
11. Start a service remotely
(Get-WmiObject -Computername Win32_Service -Filter "Name=' ' ").InvokeMethod("StopService",$null)
e.g.
(Get-WmiObject -Computername fireserver Win32_Service -Filter "DisplayName='Computer Browser'").InvokeMethod("StopService",$null)
12. Copy files
Copy-Item c:\mydoc\text.doc c:\backup
13. Create file/folder
New-Item c:\scripts\Windows PowerShell -type directory
14. Check file exist
Test-Path c:\scripts\ text.doc
15. Write data into xml file
Get-Process | Export-Clixml c:\scripts\process-list.xml
16. Run a program. Use Invoke-Expression to run a Powershell script.
Invoke-Item notepad.
17. How to see all previous commands and batch it up?
Get-History
or
h // alias for Get-History
Running it in batch
Invoke-History 34;Invoke-History 23
18. Filter command result by its property name
Get-Process -ProcessName svch* // list process with name starts with svch
Get-Process -ProcessName s?c* // list process with name starts with s followed by any character; followed by c and etc.
19. List environment variables
$env:computername
$env:classpath
More to come ....
Acronyms:
* cmdlet, wmi.
Windows PowerShell is a command-line shell and scripting environment that brings the power of the .NET Framework to command-line users and script writers. It introduces a number of powerful new concepts that enables you to extend the knowledge you have gained and the scripts you have created within the Windows Command Prompt and Windows Script Host environments.
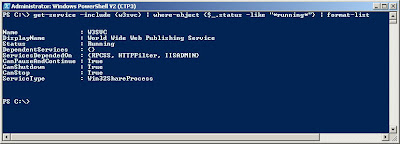
Figure 1.0 PowerShell window.
Windows PowerShell V2 Community Technology Preview 3 (CTP3)
- Download from here
- Getting Started
- Scripting with PowerShell
- Online help
- How to install/uninstall Powershell
- PowerShell Developer Guide
PowerShell Benefits
- Extension to command line windows.
- Performing common system administration tasks. E.g. managing the registry, services, processes, and event logs, and using Windows Management Instrumentation(WMI).
- Simplified, command-based navigation of the operating system, which lets users navigate the registry and other data stores
- Read WMI object values and invoke its method directly.
I hope the following list of examples could get you playing:
1. Get help on particular command: man
2. See a list of command available: get-command
3. Clear the screen: clear-host
4. Show info for Http Web Server service: get-service -include {w3svc} | where-object {$_.status -like "*running*"} | format-list (As in Figure 1.0 command)
5. Get the system date: get-date
6. Get the service DisplayName only: get-service w3svc | format-list -property DisplayName
7. List service : get-service | where-object {$_.name -like "[v-z]*"} | Sort -descending "Status"
8. Show .net component methods:
9. Get newest 5 system event log entry:
"httpd"| Get-Service -computername fireserver | Get-Member
11. Start a service remotely
(Get-WmiObject -Computername
e.g.
(Get-WmiObject -Computername fireserver Win32_Service -Filter "DisplayName='Computer Browser'").InvokeMethod("StopService",$null)
12.
Copy-Item c:\mydoc\text.doc c:\backup
13. Create file/folder
New-Item c:\scripts\Windows PowerShell -type directory
14. Check file exist
Test-Path c:\scripts\
15. Write data into xml file
Get-Process | Export-Clixml c:\scripts\process-list.xml
16. Run a program. Use Invoke-Expression
Invoke-Item notepad.
17. How to see all previous commands and batch it up?
Get-History
or
h // alias for Get-History
Running it in batch
Invoke-History 34;Invoke-History 23
18. Filter command result by its property name
Get-Process -ProcessName svch* // list process with name starts with svch
Get-Process -ProcessName s?c* // list process with name starts with s followed by any character; followed by c and etc.
19. List environment variables
$env:computername
$env:classpath
More to come ....
Acronyms:
* cmdlet, wmi.
Monday, April 20, 2009
How to find out current logon username and domain (via dos command)
- Open command prompt window (by typing in cmd in Start-> Run textbox).
- Type echo %userdomain%\%username% and press enter.
Monday, March 30, 2009
Free Regular Expression Designer
Check out this unicode regex editor from RadSoftware. Came across this in Microsoft TechNet April 2009.
Alternatively, this is the one I personally prefer -> Expresso Regex editor (version 3.0)
Alternatively, this is the one I personally prefer -> Expresso Regex editor (version 3.0)
DataView sorting issue on new record inserted
1. Scenario:
I was working on a project of feeding live data to a datagrid view (dgv) with just-in-time data loading. The dgv is populated from a datatable.DefaultView and should sort by the latest updates.
The screen data is cached and loaded from the underlying datatable when the user scrolls down the dgv. The gridview doesn't have to load 500,000 records when it initialise. Thus, greatly reducing the memory footprint and waiting time.
MSDN: Just-In-Time Data Loading in DataGridView Control
The gridview refreshes itself when new data arrives. The user sees the latest record appearing on top of the gridview.
## Code snippet (Starts)-----------------------------------------------------
DataView _latestPriceView = _dtPrices.DefaultView;
_latestPriceView.Sort = "PriceUID DESC"
// obtain the latest price unique-id and perform further processing
uid = (_latestPriceView.Table.Rows(0).Item(0))
## Code snippet (Ends)-----------------------------------------------------
2. What is the problem:
The issue happens new price arrives. It has been added to the bottom of dtPrices datatable. The dataview that build from the datatable should have sorted the latest record to the top. However, it hasn't and the row is at the bottom of the dataview rows.
What is going on here?
3. Mitigation:
The reason is dataTable is not sorted but DataView is. Finding the top element from dataview gives you the latest uid. Performing that on the unsorted datatable give you latest uid since it was retreive from data source. The latest record is at the bottom of the datatable.
Do not use theDataView.Table. Rows[0]. Reference the dataview item directly without using the table properties.
e.g.
DataView[i].Item[0]
## Code snippet (Starts)-----------------------------------------------------
DataView _latestPriceView = _dtPrices.DefaultView;
_latestPriceView.Sort = "[PriceUID] DESC"
// obtain the latest price unique-id (normally the first row from the dataview rows)
// Perform further processing ...
int32 uid = _latestPriceView(0).Item(0) // direct referencing to the view item does the trick.
If you try to use
int32 uid2 = _lastestPriceView.Table.Rows[0].Item[0] // This correspong to the datatable first row [_dtPrices(0)] rather than the last update which is inserted at the bottom of the datatable -> _dtPrices.Rows[_dtPrices.Rows.Count-1].Item[0]
## Code snippet (Ends)-----------------------------------------------------
I was working on a project of feeding live data to a datagrid view (dgv) with just-in-time data loading. The dgv is populated from a datatable.DefaultView and should sort by the latest updates.
The screen data is cached and loaded from the underlying datatable when the user scrolls down the dgv. The gridview doesn't have to load 500,000 records when it initialise. Thus, greatly reducing the memory footprint and waiting time.
MSDN: Just-In-Time Data Loading in DataGridView Control
The gridview refreshes itself when new data arrives. The user sees the latest record appearing on top of the gridview.
## Code snippet (Starts)-----------------------------------------------------
DataView _latestPriceView = _dtPrices.DefaultView;
_latestPriceView.Sort = "PriceUID DESC"
// obtain the latest price unique-id and perform further processing
uid = (_latestPriceView.Table.Rows(0).Item(0))
## Code snippet (Ends)-----------------------------------------------------
2. What is the problem:
The issue happens new price arrives. It has been added to the bottom of dtPrices datatable. The dataview that build from the datatable should have sorted the latest record to the top. However, it hasn't and the row is at the bottom of the dataview rows.
What is going on here?
3. Mitigation:
The reason is dataTable is not sorted but DataView is. Finding the top element from dataview gives you the latest uid. Performing that on the unsorted datatable give you latest uid since it was retreive from data source. The latest record is at the bottom of the datatable.
Do not use the
e.g.
## Code snippet (Starts)-----------------------------------------------------
DataView _latestPriceView = _dtPrices.DefaultView;
_latestPriceView.Sort = "[PriceUID] DESC"
// obtain the latest price unique-id (normally the first row from the dataview rows)
// Perform further processing ...
int32 uid = _latestPriceView(0).Item(0) // direct referencing to the view item does the trick.
If you try to use
int32 uid2 = _lastestPriceView.Table.Rows[0].Item[0] // This correspong to the datatable first row [_dtPrices(0)] rather than the last update which is inserted at the bottom of the datatable -> _dtPrices.Rows[_dtPrices.Rows.Count-1].Item[0]
## Code snippet (Ends)-----------------------------------------------------
Sunday, March 01, 2009
TRAC Cheat sheet
Create new project
Add user permission
- >trac-admin "C:\Trac\
" initenv - mysql connection string - mysql://root:myroot@
:3306/
Add user permission
- >trac-admin "c:\trac\
" permission add TRAC_ADMIN
Tuesday, February 24, 2009
Teamviewer - Free Remote Desktop apps
Cost: Free for non-commercial use.
For more information, go to TeamViewer website.
Scenario:
Bob tries to remotely view/control Susan's machine for virus troubleshooting.
What to do?
For Susan
- Download the TeamViewerQS and run it (Click on the link to download).
- Tell Bob the session id and password (depicted as the screen below).
- Download TeamViewer (full version) and run it.
- Connect using the session id and password provided by Susan.
Wednesday, February 18, 2009
C# How to parse date string into UK date format
DateTime ukdatetime = DateTime.Parse(usdatetime, System.Globalization.CultureInfo.CreateSpecificCulture("en-GB"));
Oracle PL/SQL SubQuery example
The following subquery uses EXISTS method to check whether the employee work in UK site. The crucial part of the query is the use of the usr.idemployee (main query) to filter the data in the subquery.
The subquery could be selecting from any tables but the query result is appended to the main query. The subquery result doesn't have to exist in any table.
select usr.iduser, usr.name,
DECODE ((SELECT 1
FROM DUAL
WHERE EXISTS (
SELECT dummy
FROM empworkplace wrk
WHERE usr.idemployee = wrk.idemployee
AND wrk.sitekey = 'UK'),
NULL, 0,
1
) AS fromUKSite,
from users usr.
The subquery could be selecting from any tables but the query result is appended to the main query. The subquery result doesn't have to exist in any table.
select usr.iduser, usr.name,
DECODE ((SELECT 1
FROM DUAL
WHERE EXISTS (
SELECT dummy
FROM empworkplace wrk
WHERE usr.idemployee = wrk.idemployee
AND wrk.sitekey = 'UK'),
NULL, 0,
1
) AS fromUKSite,
from users usr.
Subscribe to:
Posts (Atom)