How could you automate web application screen testing?
- Write unit test in Selenium or WatiN (pronounce what-in).Selenium has action replay feature and Firefox plugin to record a series of steps then generating the script for you. However, my example would be using WatiN as it's lightweight and the project dependency is only 1 library (WatiN.Core). Simple.
The following code demonstrates a unit test script to login to Google account (via Internet Explorer).
1. Download WatiN from here. Extract the WatiN.Core and add this lib to your project reference.
2. Add a test method as below. Fill in a valid Email and Passwd (replace me.gmail.com and xxx).
[TestMethod]
public void LoginGoogle()
{
using(IE _ie = new IE("https://www.google.com/accounts/ServiceLogin?service=mail&passive=true&rm=false&continue=http%3A%2F%2Fmail.google.com%2Fmail%2F%3Fui%3Dhtml%26zy%3Dl&bsv=1eic6yu9oa4y3&scc=1<mpl=default<mplcache=2"))
{
_ie.TextField(tf => tf.Name.Equals("Email")).TypeText("me@gmail.com");
_ie.TextField(tf => tf.Name.Equals("Passwd")).TypeText("xxx");
_ie.Button(Find.ByValue("Sign in")).Click();
_ie.Link(Find.ByText("Sign out")).Click();
_ie.Close();
}
{
_ie.TextField(tf => tf.Name.Equals("Email")).TypeText("me@gmail.com");
_ie.TextField(tf => tf.Name.Equals("Passwd")).TypeText("xxx");
_ie.Button(Find.ByValue("Sign in")).Click();
_ie.Link(Find.ByText("Sign out")).Click();
_ie.Close();
}
}
3. Right click on the TestMethod and click Run Tests. Result as follow (click on picture to enlarge) :
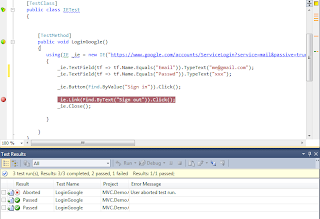
4. What you might ask. How do I find out the textbox's name for the test page?
You could use Firefox Firebug or IE7 Developer Tool to find that out.
No comments:
Post a Comment