I have tried the following:
1) Create a exe and run Process.start(
2) Create vbs and add into Window installer custom actions. Result = failed because the installer run the script before the files is installed to the target folder.
Solution:
1) Create the Window Installer project in Visual Studio2003 as normal.
2) Create a c# or vb.net DLL project. Add a new item 'Installer Class' under the project. This will create a class that we can override any default installation process.
3) Add in the following code to overwrite installation event. Remember, you have to overwrite OnAfterInstall().
protected override
{ base.OnAfterInstall (savedState);
// Add your custom code after this
// Process.Start (@"c:\mybatch.bat");
}
4) Add the new DLL project to your solution.
5) Add the newly build DLL output to the custom action under 'Install' and 'Commit' folder.
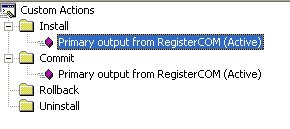
6) Compile the setup project and voilĂ , your batch file is run as soon as the installation committed.
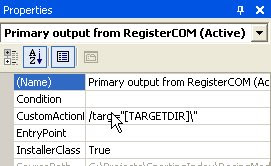
Notes:
- If you wish to pass your target directory to the Installer class.
Add the following targ= "[TARGETDIR\]" to the custom action 'CustomActionData' properties.
- How do I read the custom action parameter from the Installer class?
use the following code to read the passed in parameter.
this.Context.Parameters["targ"];
- If you want to see all the Installer Context parameters, use the following code:
Import the following namespace in your project and paste the following code.
System.Text and System.Collection.Specialized.
private void ShowContext(string where)
{
StringBuilder sb = new StringBuilder();
StringDictionary myStringDictionary = this.Context.Parameters;
sb.Append("From " + where + "\n");
if (this.Context.Parameters.Count > 0)
{
foreach (string myString in this.Context.Parameters.Keys)
{
sb.AppendFormat("String={0} Value= {1}\n", myString,
this.Context.Parameters[myString]);
}
}
MessageBox.Show(sb.ToString());
}
1 comment:
This post is very useful, I followed it step by step and succeeded!
One thing need to mention is:
targ= "[TARGETDIR\]"
is actually:
/targ="[TARGETDIR]\"
Post a Comment