What if you want to validate a string with a floating point number without any other characters in it except minus (-) sign. You can use multiple if/switch statement but the code goes long way down
to cater for all invalid characters (such as * & $ £).
Regular Expression comes to the rescue. Lets take the following example.
e.g
Valid numbers
0.45, -0.33, -34, 100, 0, -3.44
Invalid numbers
$3, +6.55, --56, 46*, 33"!, &, +88
Regular Expression = (^-?\d{1,3}\.?\d{0,5}?$)
Explanations:
^-? == ^ sign means match the string starting with. ? (question mark) means optional. The string means match the string that starts with an optional minus sign. This mean string starts with "-2" and "2" are valid. "+2", "@%£2" are invalid.
Monday, August 06, 2007
Thursday, August 02, 2007
How to programatically open acrobat reader?
If you do not want to open acrobat pdf reader to show your pdf. You can always display Acrobat Reader from Internet Explorer. The following code snippet is done in C#. Import System.Diagnostics namespace in order to use the Process class.
Process.Start(@"C:\Program Files\Internet Explorer\iexplore.exe", @"file://Help.pdf")
or
// This will use the the default registered application to launch the document
Process.Start( @"file://Help.pdf")
Process.Start(@"C:\Program Files\Internet Explorer\iexplore.exe", @"file://Help.pdf")
or
// This will use the the default registered application to launch the document
Process.Start( @"file://Help.pdf")
How to run batch file using Visual Studio Window Installer?
I have recently came across a problem with my VS.net2003 Window Installer (.net fx 1.1). I have added some dll components to the setup package. I wish to run a batch file after the installation is completed. How do I achieve that?
I have tried the following:
1) Create a exe and run Process.start() and add it as custom actions. Result = failed.
2) Create vbs and add into Window installer custom actions. Result = failed because the installer run the script before the files is installed to the target folder.
Finally, I found the problem lies in the where you overwrite the System.Configuration.Install namespace events. We should use OnAfterInstall() rather than OnInstall() or OnCommit().
Solution:
1) Create the Window Installer project in Visual Studio2003 as normal.
2) Create a c# or vb.net DLL project. Add a new item 'Installer Class' under the project. This will create a class that we can override any default installation process.
3) Add in the following code to overwrite installation event. Remember, you have to overwrite OnAfterInstall().
protected override void OnAfterInstall(IDictionary savedState)
{ base.OnAfterInstall (savedState);
// Add your custom code after this
// Process.Start (@"c:\mybatch.bat");
}
4) Add the new DLL project to your solution.
5) Add the newly build DLL output to the custom action under 'Install' and 'Commit' folder. 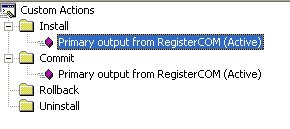
6) Compile the setup project and voilĂ , your batch file is run as soon as the installation committed.
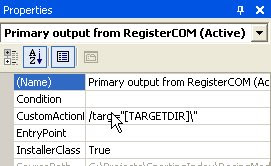
Notes:
- If you wish to pass your target directory to the Installer class.
Add the following targ= "[TARGETDIR\]" to the custom action 'CustomActionData' properties.
- How do I read the custom action parameter from the Installer class?
use the following code to read the passed in parameter.
this.Context.Parameters["targ"];
- If you want to see all the Installer Context parameters, use the following code:
Import the following namespace in your project and paste the following code.
System.Text and System.Collection.Specialized.
private void ShowContext(string where)
{
StringBuilder sb = new StringBuilder();
StringDictionary myStringDictionary = this.Context.Parameters;
sb.Append("From " + where + "\n");
if (this.Context.Parameters.Count > 0)
{
foreach (string myString in this.Context.Parameters.Keys)
{
sb.AppendFormat("String={0} Value= {1}\n", myString,
this.Context.Parameters[myString]);
}
}
MessageBox.Show(sb.ToString());
}
I have tried the following:
1) Create a exe and run Process.start(
2) Create vbs and add into Window installer custom actions. Result = failed because the installer run the script before the files is installed to the target folder.
Solution:
1) Create the Window Installer project in Visual Studio2003 as normal.
2) Create a c# or vb.net DLL project. Add a new item 'Installer Class' under the project. This will create a class that we can override any default installation process.
3) Add in the following code to overwrite installation event. Remember, you have to overwrite OnAfterInstall().
protected override
{ base.OnAfterInstall (savedState);
// Add your custom code after this
// Process.Start (@"c:\mybatch.bat");
}
4) Add the new DLL project to your solution.
5) Add the newly build DLL output to the custom action under 'Install' and 'Commit' folder.
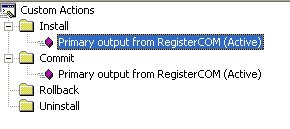
6) Compile the setup project and voilĂ , your batch file is run as soon as the installation committed.
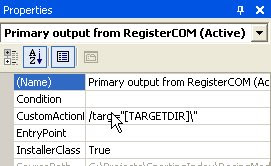
Notes:
- If you wish to pass your target directory to the Installer class.
Add the following targ= "[TARGETDIR\]" to the custom action 'CustomActionData' properties.
- How do I read the custom action parameter from the Installer class?
use the following code to read the passed in parameter.
this.Context.Parameters["targ"];
- If you want to see all the Installer Context parameters, use the following code:
Import the following namespace in your project and paste the following code.
System.Text and System.Collection.Specialized.
private void ShowContext(string where)
{
StringBuilder sb = new StringBuilder();
StringDictionary myStringDictionary = this.Context.Parameters;
sb.Append("From " + where + "\n");
if (this.Context.Parameters.Count > 0)
{
foreach (string myString in this.Context.Parameters.Keys)
{
sb.AppendFormat("String={0} Value= {1}\n", myString,
this.Context.Parameters[myString]);
}
}
MessageBox.Show(sb.ToString());
}
Saturday, June 16, 2007
Oriental Fire

I definitely recommend the following:
- 111. DakGangJung
- 109. Chicken and Sweet Egg domburi
- 79. Chicken Bulgogi
- Green Tea ice cream
Address
19 Ashley Road
Altrinham WA14 2DP
Cheshire
Great Britain
Tel: 0161-9411-550
Website: http://www.orientalfire.co.uk/
Google map
Oriental Fire
Thursday, February 22, 2007
Nant script map/un-map network drive automatically
How do we map network drive automatically using Nant script?
Use the exec task with net.exe command.
The following example attempts to map drive P: to network location = \\192.168.12.1\c$ using domain = testDomain and domainUserLogin = userid with password = bob.
The " is to enclosed the network path and the password.
<exec program="net" commandline="use P:$html_quot$\\192.168.12.1\c$ $html_quot$ /user:testDomain\userid $html_quot$bob$html_quot$ ">
</exec>
The actual dos command to map network drive look like this:
net use P: "\\192.168.12.1\c$" /user:testDomain\userid "bob"
How to disconnect the network drive then?
Use the following dos command to disconnect drive P :
net use P: /DELETE
Nant script equivalent:
<exec program="net" commandline="use P: /DELETE">
What if we want to verify the map drive existed?
<if test="${directory::exists('P:')}">
<exec program="net" commandline="use P: /DELETE "/>
</if>
Dos command reference [net use] to map network drive
Use the exec task with net.exe command.
The following example attempts to map drive P: to network location = \\192.168.12.1\c$ using domain = testDomain and domainUserLogin = userid with password = bob.
The " is to enclosed the network path and the password.
<exec program="net" commandline="use P:$html_quot$\\192.168.12.1\c$ $html_quot$ /user:testDomain\userid $html_quot$bob$html_quot$ ">
</exec>
The actual dos command to map network drive look like this:
net use P: "\\192.168.12.1\c$" /user:testDomain\userid "bob"
How to disconnect the network drive then?
Use the following dos command to disconnect drive P :
net use P: /DELETE
Nant script equivalent:
<exec program="net" commandline="use P: /DELETE">
What if we want to verify the map drive existed?
<if test="${directory::exists('P:')}">
<exec program="net" commandline="use P: /DELETE "/>
</if>
Dos command reference [net use] to map network drive
Wednesday, February 14, 2007
Hide Office document Design mode
When you open a Ms Office Excel, Word document that has embedded controls (combo box, check box and etc) , it always shows the Design toolbar. This is very annoying.
I have 'googled' for answer and no working solution found. I decided to attempt it myself and it works. However, it requires macro execution coz i did it using VBA.
I have tried VBA ActiveDocument.ToggleFormsDesign . Doesn't work.
Solution:
- Open the office document.
- Open the VBA Editor (Alt-F11).
- Add the following to the document_open events. Double click ThisDocument (under Microsoft Word Object).
- Save the document.
- Voila. Done.
I have 'googled' for answer and no working solution found. I decided to attempt it myself and it works. However, it requires macro execution coz i did it using VBA.
I have tried VBA ActiveDocument.ToggleFormsDesign . Doesn't work.
Solution:
- Open the office document.
- Open the VBA Editor (Alt-F11).
- Add the following to the document_open events. Double click ThisDocument (under Microsoft Word Object).
- Exit VBA Editor.
Private Sub Document_Open()
CommandBars("Control Toolbox").Visible = False
CommandBars("Exit Design Mode").Visible = False
End Sub
- Save the document.
- Voila. Done.
Subscribe to:
Posts (Atom)